Getting Device Status
By clicking on a specific device in the device list, users can navigate to its control interface, which displays different information according to device types.
This section takes the control interface of light bulbs as an example, which contains the bulb's name, power on/off status, brightness, hue, and saturation. This information was obtained eariler when getting the list of bound devices in Section 10.6.2. The control interface for light bulbs is shown in Figure 10.29.
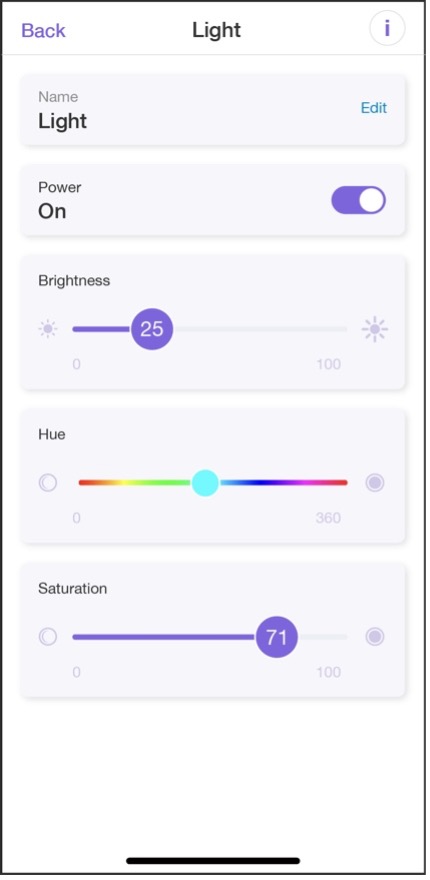
Given that one device might be controlled by different users, we should keep the device information in the smartphone app up to date by regularly refreshing and getting device status.
The API to get the status of a device is shown in Figure 10.30 and can be found at https://swaggerapis.rainmaker.espressif.com/#/Node%20Parameter%20Operations/getnodestate.

GET /v1/user/nodes/params?node_id=string
Authorization: $accesstoken
In response to the "get status" request, the server returns:
{
"Light": {
"brightness": 0,
"output": true
},
"Switch": {
"output": true
}
}
Among the returned fields, Light
represents the brightness of the device, and brightness
is the specific value; Switch
represents the on/off status of the device.
Getting the device's status in Android
📝 Source code
For the source code of getting device status in Android, please refer to
book-esp32c3-iot-projects/phone_app/app_android/app/src/main/java/com/espressif/cloudapi/ApiManager.java
.
public void getParamsValues(final String nodeId, final ApiResponseListener listener) {
apiInterface.getParamValue(AppConstants.URL_USER_NODES_PARAMS,
accessToken, nodeId).enqueue(new Callback<ResponseBody>() {
@Override
public void onResponse(Call<ResponseBody> call, Response<ResponseBody> response) {
//Code Omitted
}
@Override
public void onFailure(Call<ResponseBody> call, Throwable t) {
t.printStackTrace();
listener.onNetworkFailure(new Exception(t));
}
});
}
Getting the device's status in iOS
📝 Source code
For the source code of getting device status in iOS, please refer to
book-esp32c3-iot-projects/phone_app/app_ios/ESPRainMaker/ESPRainMaker/AWSCognito/ESPAPIManager.swift
.
func getDeviceParams(device: Device, completionHandler: @escaping (ESPNetworkError?) -> Void) {
ESPExtendUserSessionWorker().checkUserSession(){accessToken, error in
if let token = accessToken {
let headers: HTTPHeaders = ["Content-Type": "application/json",
"Authorization": token]
let url = Constants.setParam + "?node_id=" + (device.node?.node_id ?? "")
self.session.request(url, method: .get, parameters: nil, encoding: JSONEncoding.default,
headers: headers).responseJSON { response in
//Code Omitted
}
} else {
if self.validatedRefreshToken(error: error) {
completionHandler(.emptyToken)
}
}
}
}